1. Basics of Bash
What is Bash Shell?
Bash (Bourne Again Shell) is the most commonly used command-line interface in Linux distributions. This simple yet powerful tool provides a platform for users to interact with the system, allowing them to perform essential tasks such as file operations, program execution, and task management.
Advantages of Bash
- Powerful scripting capabilities: Bash allows users to automate complex tasks using shell scripts.
- Extensive support: It is supported by most Unix-based operating systems and Linux distributions.
- High customizability: Users can customize their environment according to their workflow using aliases and shell functions.
# Simple Bash command example
echo "Hello, World!"
2. Basic Bash Commands
File Operations
Here are some of the most commonly used file operation commands in Bash.
ls
: Lists the contents of a directory.cd
: Changes the directory.cp
: Copies files or directories.mv
: Moves or renames files.rm
: Deletes files.
# Display detailed contents of a directory
ls -l
# Move to the home directory
cd ~
# Copy a file
cp source.txt destination.txt
# Move a file
mv old_name.txt new_name.txt
# Delete a file
rm unwanted_file.txt
System Information and Process Management
Checking system information and managing processes are also essential tasks.
ps
: Displays active processes.top
: Shows a real-time list of processes and system overview.kill
: Sends a signal to terminate a process.
# Display active processes
ps aux
# Show system overview and process list
top
# Terminate process with ID 1234
kill 1234
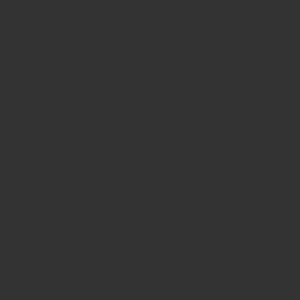
3. Writing Bash Scripts
Basic Script Structure
A Bash script is a file containing multiple commands. By creating scripts, you can automate and execute a series of operations.
#!/bin/bash
# This line is called a shebang and specifies the shell interpreter for the script.
echo "Hello, World!" # Displays a string using the echo command
Using Variables
Variables can be used to store and reuse data within a script.
#!/bin/bash
message="Hello, Bash Scripting!"
echo $message
Conditional Statements and Loops
Conditional statements and loops help handle complex logic and repetitive tasks.
#!/bin/bash
# Example of an if statement
if [ $1 -gt 100 ]
then
echo "The number is greater than 100."
else
echo "The number is 100 or less."
fi
# Example of a for loop
for i in 1 2 3 4 5
do
echo "Looping ... number $i"
done
4. Automating Tasks with Bash
Overview of Task Automation
By using Bash scripts, you can efficiently automate routine tasks such as system backups, data synchronization, and report generation, reducing the effort required for system administration.
Automated Backup Script
For daily data protection, the following script regularly backs up a specified directory.
#!/bin/bash
SRC_DIR="/home/user/documents"
DST_DIR="/backup/documents"
DATE=$(date +%Y%m%d)
# Create the backup directory if it doesn't exist
if [ ! -d "$DST_DIR" ]; then
mkdir -p "$DST_DIR"
fi
# Compress and back up the directory contents
tar -czf "$DST_DIR/backup_$DATE.tar.gz" -C "$SRC_DIR" .
echo "Backup completed successfully."
Running Scripts Automatically with cron Jobs
Using cron, you can schedule the above backup script to run daily at 2 AM.
0 2 * * * /path/to/backup.sh
Error Handling and Notifications
To handle errors during the backup process, add error detection and send notifications to the administrator when issues occur.
#!/bin/bash
SRC_DIR="/home/user/documents"
DST_DIR="/backup/documents"
LOG_FILE="/var/log/backup.log"
DATE=$(date +%Y%m%d)
if [ ! -d "$DST_DIR" ]; then
mkdir -p "$DST_DIR"
fi
if tar -czf "$DST_DIR/backup_$DATE.tar.gz" -C "$SRC_DIR" .; then
echo "Backup successful on $DATE" >> $LOG_FILE
else
echo "Backup failed on $DATE" | mail -s "Backup Failure" admin@example.com
fi
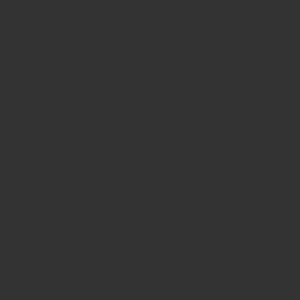
5. Troubleshooting and Common Errors
Understanding and Handling Bash Errors
It is common to encounter errors when executing Bash scripts. This section explains some common errors and how to resolve them.
Command Not Found Error
This error occurs when the command you are trying to execute is not installed on the system or the path is not set correctly.
command not found
- Solution: Check if the command is installed and ensure that the
$PATH
environment variable is correctly set.
Permission Denied Error
This error occurs when you lack the necessary permissions to access a file or directory.
Permission denied
- Solution: Execute the operation with an appropriate user account or change the file permissions using the
chmod
orchown
commands.
Syntax Error
This error happens when there is an incorrect syntax in the script.
syntax error: unexpected end of file
- Solution: Carefully review the script and fix any syntax errors.
File Not Found Error
This error occurs when the specified file does not exist.
No such file or directory
- Solution: Verify that the file path is correct and ensure that the file exists.
Using Debugging Tools
To debug Bash scripts, use set -x
, which displays each command step as it is executed, making it easier to identify errors.
set -x # Enable script debugging
6. Advanced Bash Techniques
Working with Functions
Functions in Bash scripts help modularize code and make it reusable.
#!/bin/bash
# Define a function
greet() {
echo "Hello, $1!"
}
# Call the function with an argument
greet "Alice"
Handling User Input
Bash scripts can interact with users by accepting input from the command line.
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name!"
Working with Arrays
Bash supports arrays, which allow you to store multiple values in a single variable.
#!/bin/bash
fruits=("Apple" "Banana" "Cherry")
# Accessing elements
echo "First fruit: ${fruits[0]}"
# Looping through an array
for fruit in "${fruits[@]}"; do
echo "Fruit: $fruit"
done
Regular Expressions and String Manipulation
Bash scripts can manipulate strings and match patterns using regular expressions.
#!/bin/bash
text="Bash scripting is fun!"
# Extract a substring
echo "${text:0:4}" # Output: Bash
# Replace a word
echo "${text//fun/exciting}" # Output: Bash scripting is exciting!
7. Conclusion
Summary
This guide covered everything from basic Bash commands to scripting, automation, and troubleshooting. By mastering Bash, you can efficiently manage and automate system tasks.
Further Learning
- Explore advanced scripting techniques such as process substitution and named pipes.
- Learn about integrating Bash with other programming languages like Python.
- Experiment with real-world automation tasks to enhance your scripting skills.
By continuously improving your Bash skills, you can become a more proficient system administrator or developer.