- 1 1. Introduction
- 2 2. Installing Docker on Ubuntu
- 3 3. Basic Operations with Docker Images
- 4 4. Creating Custom Docker Images with Dockerfile
- 5 5. Configuring the Japanese Language Environment in an Ubuntu Container
- 6 6. Optimizing and Reducing the Size of Docker Images
- 7 7. Practical Guide: Developing Applications in an Ubuntu Container
- 8 8. FAQ & Troubleshooting
1. Introduction
What is Docker?
Docker is a platform that utilizes container-based virtualization technology to efficiently develop, distribute, and run applications. Unlike traditional virtual machines (VMs), containers share the host OS kernel, allowing for faster startup times and lower resource consumption.
Benefits of Using Docker on Ubuntu
Ubuntu is one of the Linux distributions highly compatible with Docker. The key benefits include:
- Official Support: Docker officially supports Ubuntu, and installation is easy via the official repository.
- Stable Package Management: Ubuntu’s APT package manager makes it easy to manage Docker versions.
- Extensive Community Support: Ubuntu has a large global user base, making it easier to find solutions when troubleshooting issues.
What You Will Learn in This Article
This article will guide you through the following topics step by step:
- How to install Docker on Ubuntu
- Basic Docker image operations
- Creating custom images using Dockerfile
- Configuring the Japanese language environment in an Ubuntu container
- Optimizing and reducing the size of Docker images
- Developing applications in an Ubuntu container
- Common errors and troubleshooting solutions
This guide is useful for both beginners and advanced users, so feel free to use it as a reference.
2. Installing Docker on Ubuntu
Installing Docker Using the Official Repository
On Ubuntu, you can easily install Docker using the official repository. Follow the steps below to set it up.
1. Remove Existing Docker Packages
Ubuntu provides a package called docker.io
by default, but it may be an outdated version. It’s recommended to remove it first.
sudo apt remove docker docker-engine docker.io containerd runc
2. Install Required Packages
Before installing Docker, install the necessary dependency packages.
sudo apt update
sudo apt install -y apt-transport-https ca-certificates curl software-properties-common
3. Add the Official Docker Repository
Add Docker’s official GPG key and set up the repository.
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
echo "deb [arch=$(dpkg --print-architecture) signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
4. Install Docker
Once the repository is added, install Docker.
sudo apt update
sudo apt install -y docker-ce docker-ce-cli containerd.io
5. Verify the Installation
To check if Docker has been installed correctly, display the version information.
docker --version
Initial Setup After Installation
1. Start and Enable the Docker Service
Start the Docker service and configure it to run automatically on system startup.
sudo systemctl start docker
sudo systemctl enable docker
2. Allow Non-root Users to Use Docker
By default, only the root user can run Docker. To allow a regular user to use Docker commands, add the user to the docker
group.
sudo usermod -aG docker $USER
To apply the changes, log out and log back in.
3. Test Docker
Run the hello-world
container to check if Docker is working correctly.
docker run hello-world
If you see the message “Hello from Docker!”, the installation was successful.
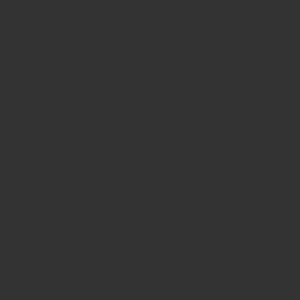
3. Basic Operations with Docker Images
What is a Docker Image?
A Docker image serves as a template for creating containers. By using an Ubuntu-based Docker image, you can quickly set up an Ubuntu environment within a container.
Downloading Ubuntu Images from Docker Hub
Docker Hub hosts a vast collection of official Docker images. To download an Ubuntu image, use the following command:
docker pull ubuntu
Starting and Stopping Containers
You can start a container using the downloaded Ubuntu image with the following command:
docker run -it ubuntu bash
This command opens a shell within the Ubuntu container, allowing you to interact with it directly.
Listing Running Containers
To check which containers are currently running, use this command:
docker ps
To display all containers, including stopped ones, add the -a
option:
docker ps -a
Stopping and Removing Containers
To stop a running container, use the following command:
docker stop [container ID or name]
To remove an unnecessary container, use this command:
docker rm [container ID or name]
Managing Docker Images
To list all downloaded Docker images, use the following command:
docker images
To remove an unused image, use this command:
docker rmi [image ID]
4. Creating Custom Docker Images with Dockerfile
What is a Dockerfile?
A Dockerfile is a configuration file used to create Docker images. By defining specific instructions in a Dockerfile, you can build customized Docker images tailored to your needs. This helps standardize development environments and include necessary packages in the image.
Basic Syntax of a Dockerfile
A Dockerfile typically includes the following key commands:
Command | Description |
---|---|
FROM | Specifies the base image |
RUN | Executes commands to build the image |
COPY | Copies files into the container |
WORKDIR | Sets the working directory |
CMD | Defines the default command to run when the container starts |
ENTRYPOINT | Specifies the main command executed when the container runs |
Creating a Custom Ubuntu-Based Docker Image
Follow these steps to create a custom Docker image based on Ubuntu.
1. Create a Working Directory
First, create a new project directory and navigate into it.
mkdir my-ubuntu-image
cd my-ubuntu-image
2. Create a Dockerfile
Inside the directory, create a Dockerfile
and add the following content:
# Use the official Ubuntu base image
FROM ubuntu:latest
# Maintainer information (optional)
LABEL maintainer="your-email@example.com"
# Update package lists and install basic tools
RUN apt update && apt install -y curl vim git
# Set the working directory
WORKDIR /workspace
# Default command executed when the container starts
CMD ["bash"]
3. Build the Docker Image
Use the following command to build the custom image from the Dockerfile.
docker build -t my-ubuntu-image .
The -t
option assigns a name to the image.
4. Verify the Built Image
Check the list of available images to ensure that the new image was created successfully.
docker images
5. Run a Container from the Custom Image
Start a container using the newly created custom image.
docker run -it my-ubuntu-image
This container should include tools like curl
and vim
as specified in the Dockerfile.
5. Configuring the Japanese Language Environment in an Ubuntu Container
By default, the Ubuntu Docker image is set to English. If you need to use Japanese, additional configurations are required.
Setting Up Japanese Locale
To enable Japanese display and input in an Ubuntu container, install the necessary language packs.
1. Install Required Packages
apt update
apt install -y language-pack-ja locales
2. Configure the Locale
Set the locale and apply the settings.
locale-gen ja_JP.UTF-8
update-locale LANG=ja_JP.UTF-8
3. Apply the Settings
export LANG=ja_JP.UTF-8
Setting Up Japanese Input
To enable Japanese text input in the terminal, install ibus-mozc
.
apt install -y ibus-mozc
If you are using a GUI environment, add the following environment variables:
export GTK_IM_MODULE=ibus
export XMODIFIERS=@im=ibus
export QT_IM_MODULE=ibus
Using GUI Applications
To run GUI applications inside a Docker container, you can use an X server.
Install an X server on the host machine and run the container with X11 enabled.
docker run -e DISPLAY=$DISPLAY -v /tmp/.X11-unix:/tmp/.X11-unix my-ubuntu-image
6. Optimizing and Reducing the Size of Docker Images
Optimizing Docker images improves container startup speed and reduces storage usage. This section introduces methods for creating lightweight images.
Creating a Lightweight Ubuntu-Based Image
The default ubuntu:latest
image is relatively large. To reduce the container size, consider using a smaller alternative like ubuntu:minimal
.
FROM ubuntu:minimal
Alternatively, you can use Alpine Linux, which is significantly smaller than Ubuntu.
FROM alpine:latest
RUN apk add --no-cache bash curl
This method can reduce the image size by several hundred megabytes.
Removing Unnecessary Files to Reduce Image Size
After installing packages with apt-get
, you can remove unnecessary cache files to minimize the image size.
RUN apt update && apt install -y curl vim \
&& apt clean \
&& rm -rf /var/lib/apt/lists/*
The rm -rf /var/lib/apt/lists/*
command deletes the package list, reducing unnecessary data.
Using Multi-Stage Builds
With multi-stage builds, you can use a temporary container for building dependencies while keeping the final image lightweight.
FROM ubuntu as builder
RUN apt update && apt install -y gcc
FROM ubuntu:minimal
COPY --from=builder /usr/bin/gcc /usr/bin/gcc
This technique allows you to exclude unnecessary development tools from the final image, making it significantly smaller.
7. Practical Guide: Developing Applications in an Ubuntu Container
In this section, we will set up a development environment inside an Ubuntu container and demonstrate how to develop applications using Docker.
Setting Up a Python Development Environment
To create a Python development environment inside a Docker container, use the following Dockerfile.
FROM ubuntu:latest
RUN apt update && apt install -y python3 python3-pip
CMD ["python3"]
Build the image and run the container.
docker build -t python-dev .
docker run -it python-dev
Inside the container, you can execute the python3
command to run Python scripts and develop applications.
Setting Up a Node.js Development Environment
For Node.js development, use the following Dockerfile.
FROM ubuntu:latest
RUN apt update && apt install -y nodejs npm
CMD ["node"]
Build and run the container.
docker build -t node-dev .
docker run -it node-dev
With this setup, you can use the node
command to execute JavaScript code and develop applications in a controlled environment.
8. FAQ & Troubleshooting
While using Docker, you may encounter various issues. This section provides solutions to common problems and frequently asked questions.
Difference Between Docker and Virtual Machines
- Docker: Shares the host OS kernel, making it lightweight and enabling fast container startup.
- Virtual Machines (VMs): Each VM has its own OS, consuming more resources and taking longer to start.
Docker is more efficient in resource utilization, making it ideal for development environments and automated deployments.
Persisting Data in an Ubuntu Container
To retain data even after a container is stopped, use volume mounting.
docker run -v my_data:/data ubuntu
The my_data
volume stores data persistently, allowing it to be accessed even after the container is removed.
Common Errors and Solutions
1. permission denied
Error
If you see a permission denied
error when running Docker commands, your user might not be in the docker
group.
Add your user to the docker
group with the following command:
sudo usermod -aG docker $USER
After running this command, log out and log back in to apply the changes.
2. image not found
Error
If an image has been removed from Docker Hub, specify a different tag to pull a valid version.
docker pull ubuntu:22.04
By specifying a version, you can ensure that you download a working image.